What Is Python Programming Language – for Beginners
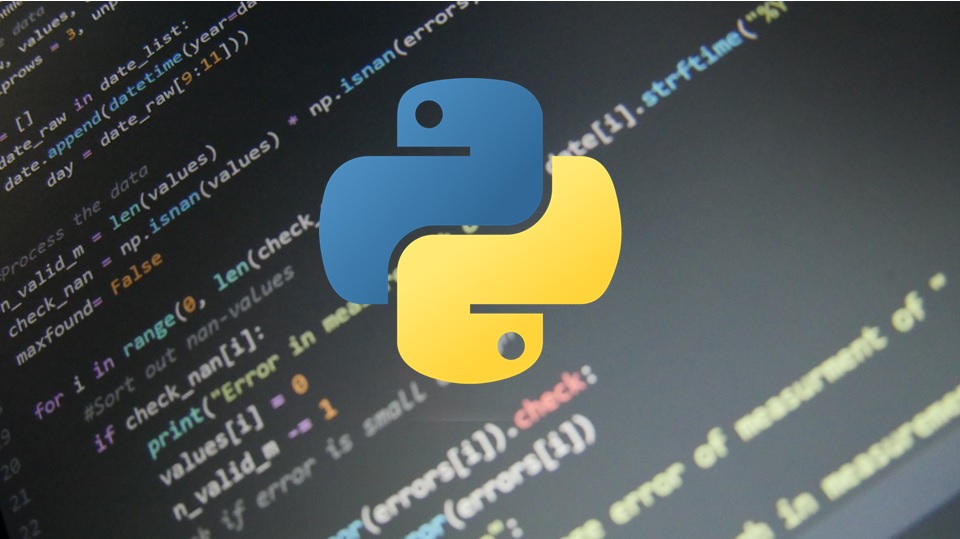
What Is Python Programming Language – for Beginners
In the vast realm of programming languages, one stands out as a versatile, powerful, and incredibly popular tool: Python. If you’re new to programming or an experienced developer looking to expand your skill set, delving into Python is a worthwhile endeavor. This article will serve as your comprehensive guide to Python, exploring its history, features, applications, and why it’s the go-to language for everything from web development to machine learning.
Table of Contents
- What is Python?
- Why Use Python?
- Python Syntax: A Beginner’s Guide
- Python in Action: Examples and Applications
- Exploring Python Software
- The Python Software Foundation: Nurturing a Community
- A Python Tutorial for Beginners
- Diving Deeper: Learning Python for Machine Learning
- The Role of Modules in Python
- Web Development with Python: A Primer
- Understanding Python Enhancement Proposals
- The History and Evolution of Python
- Frameworks and Libraries: Enhancing Python’s Power
- Features That Make Python Stand Out
- Python Compilers: Turning Code into Action
- Sharpen Your Skills: Python Exercises
- Python Servers: Powering Your Applications
What is Python?
At its core, Python is a high-level programming language with dynamic semantics. It’s often touted as one of the most readable and beginner-friendly languages, making it an excellent choice for those new to programming. Created by Guido van Rossum and first released in 1991, Python has since grown into a powerhouse in the coding world.
Python is an interpreted language, which means that code written in Python is not directly translated into machine-level instructions by the computer. Instead, it relies on an interpreter to execute the code line by line.
Why Use Python?
Easy to Learn
One of Python’s biggest draws is its simplicity. The language emphasizes readability and a clean syntax, which means developers can write code that is both concise and easy to understand. This makes Python an ideal language for beginners and experienced programmers alike.
Versatility
Python’s versatility is unmatched. It’s a general-purpose programming language, meaning it can be used for a wide range of applications, from web development to scientific computing. Whether you’re building a website, analyzing data, or developing machine learning models, Python has you covered.
Rich Ecosystem
Python boasts a vast array of libraries and frameworks that extend its capabilities even further. Need to work with data? The Pandas library has you covered. Interested in web development? Flask and Django are popular choices. Python’s rich ecosystem ensures that whatever your project entails, there’s likely a tool or library available to streamline the process.
Python Syntax: A Beginner’s Guide
Understanding Python’s syntax is crucial for writing effective code. Here are some basic syntax elements every aspiring Python programmer should know:
Indentation
In Python, indentation is not just for readability—it’s a fundamental part of the language’s syntax. Instead of using curly braces or keywords to define code blocks, Python uses indentation. This forces clean, readable code and helps maintain consistency throughout a project.
Variables and Data Types
Python is dynamically typed, meaning you don’t need to declare the type of a variable when you create one. For example:
name = "Alice" # This is a string age = 30 # This is an integer
Python will infer the type based on the value assigned to the variable.
Control Structures
Python supports common control structures like if statements, for loops, and while loops. Here’s an example of an if statement:
if age < 18: print("You are a minor") else: print("You are an adult")
Functions
Functions in Python are defined using the def
keyword. They can take parameters and return values. Here’s a simple function that calculates the square of a number:
def square(x): return x * x
To call this function, you would use square(5)
, which would return 25
.
Python in Action: Examples and Applications
Data Science
Python has become the language of choice for many data scientists due to its powerful libraries like Pandas, NumPy, and Matplotlib. These libraries make tasks such as data manipulation, analysis, and visualization efficient and straightforward.
Web Development
Frameworks like Django and Flask have made Python a popular choice for web development. Django, known for its “batteries-included” philosophy, provides a full-featured framework for building web applications, while Flask offers a lightweight and modular approach.
Machine Learning and AI
Python’s simplicity and powerful libraries, such as TensorFlow and PyTorch, have made it a dominant force in the realm of machine learning and artificial intelligence. From image recognition to natural language processing, Python is at the forefront of cutting-edge technologies.
Exploring Python Software
Python’s versatility is reflected in the wide range of software applications built using the language. Here are a few notable examples:
- YouTube: The popular video-sharing platform uses Python for various tasks, including view counting, content management, and scalability.
- Instagram: This social media giant relies on Python for its backend infrastructure. The Django framework powers many of its features.
- Dropbox: Python is at the core of Dropbox’s file storage system. The simplicity and readability of Python have been instrumental in maintaining and scaling their platform.
The Python Software Foundation: Nurturing a Community
The Python Software Foundation (PSF) is a non-profit organization that manages the open-source licensing for Python and supports the community-driven development of the language. It plays a vital role in ensuring Python remains free and accessible to all.
Getting Started with Python
Installation and Setup
Before you can start coding in Python, you need to set up your development environment. Here’s a step-by-step guide to getting started:
- Download Python: Visit the official Python website and download the latest version of Python that is compatible with your operating system. Python is available for Windows, macOS, and Linux.
- Install Python: Run the downloaded installer and follow the on-screen instructions to install Python on your computer. Make sure to select the option to add Python to your system’s PATH to easily access it from the command line.
- IDE or Text Editor: Choose an Integrated Development Environment (IDE) or a text editor to write your Python code. Some popular options include PyCharm, Visual Studio Code, and Sublime Text. These tools offer features such as code autocompletion, debugging, and project management.
Your First Python Program
Now that you have Python installed, let’s write a simple “Hello, World!” program to get started. Open your preferred IDE or text editor and follow these steps:
- Open a New File: Create a new file and save it with a
.py
extension, for example,hello_world.py
. - Write the Code: Type the following code into your file:
print("Hello, World!")
- Run the Program: Save the file and execute it by running the
hello_world.py
file. You should see the output “Hello, World!” printed in the console.
Congratulations! You’ve just written your first Python program. This simple example demonstrates the basic syntax of Python, where theprint()
function is used to display the text “Hello, World!” on the console.
Basic Syntax and Concepts
- Variables: As mentioned earlier, Python is dynamically typed, so you don’t need to declare variable types. Just assign a value:
name = "Alice" age = 30
- Lists: Lists are versatile data structures in Python that can hold a collection of items. You can create a list like this:
fruits = ["apple", "banana", "cherry"]
- Loops: For loops are handy for iterating over a sequence of elements. Here’s an example:
for fruit in fruits: print(fruit)
This will print each fruit in the fruits
list.
- Functions: We’ve already seen functions in action. Here’s another example:
def greet(name): print("Hello, " + name + "!")
You can then call this function with greet("Alice")
, which would print Hello, Alice!
.
Diving Deeper: Learning Python for Machine Learning
Machine learning is a hot topic in the tech world, and Python is the language of choice for many machine learning practitioners. If you’re interested in diving into this field, here are some key concepts and libraries to explore:
Key Libraries
- NumPy: Essential for numerical computing in Python. It provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions.
- Pandas: A powerful data manipulation library that offers data structures like DataFrames, which are ideal for handling structured data.
- Matplotlib: Used for creating static, interactive, and animated visualizations in Python.
Machine Learning Algorithms
- Scikit-learn: A library that provides simple and efficient tools for data mining and data analysis, built on NumPy, SciPy, and Matplotlib.
- TensorFlow and PyTorch: For deep learning applications, these libraries offer powerful tools and abstractions for building and training neural networks.
Example: Linear Regression
Here’s a simple example of using Python for linear regression, a fundamental machine learning algorithm:
import numpy as np
from sklearn.linear_model import LinearRegression
# Create some sample data
X = np.array([[1], [2], [3], [4]])
y = np.array([2, 4, 6, 8])
# Create a linear regression model
model = LinearRegression()
# Fit the model to the data
model.fit(X, y)
# Predict
X_new = np.array([[5]])
prediction = model.predict(X_new)
print(prediction) # Output: [10.]
In this example, we’re fitting a linear regression model to predict values based on input data.
The Role of Modules in Python
Modules in Python are files that consist of Python code. They can define functions, classes, and variables that you can use once imported into your scripts. Here’s how you can create and use a module:
- Create a Module: Save the following code in a file named
mymodule.py
:
def greeting(name):
print("Hello, " + name)
- Use the Module: In another Python script, you can import and use this module:
import mymodule
mymodule.greeting("Alice") # Output:Hello, Alice
This allows you to organize your code into reusable components.
Web Development with Python: A Primer
Python’s web development frameworks, such as Django and Flask, have made building web applications a breeze. Let’s take a brief look at each:
Django
- Features: Django is a high-level web framework known for its “batteries-included” approach. It comes with built-in features like authentication, URL routing, and an ORM (Object-Relational Mapper) for interacting with databases.
- Use Cases: Ideal for building complex, database-driven websites. Django’s admin panel, which is generated automatically, is a powerful tool for managing site content.
Flask
- Features: Flask is a lightweight and modular micro-framework. It’s highly customizable and provides the essentials for web development without imposing too much structure.
- Use Cases: Great for small to medium-sized applications, APIs, and prototypes. Flask’s simplicity makes it easy to get started with web development.
Understanding Python Enhancement Proposals
Python Enhancement Proposals (PEPs) are design documents that provide information to the Python community or describe a new feature for Python or its processes. These proposals are crucial for maintaining and evolving the Python language. Some notable PEPs include:
- PEP 8: Style Guide for Python Code. It provides guidelines for formatting Python code to improve its readability.
- PEP 20: The Zen of Python. This is a collection of guiding principles for writing computer programs in Python. It emphasizes readability and simplicity.
The History and Evolution of Python
Python’s journey from its inception to its current status as one of the most popular programming languages is a fascinating tale. Here are some key milestones:
- 1989: Guido van Rossum begins working on Python at the National Research Institute for Mathematics and Computer Science in the Netherlands.
- 1991: Python 0.9.0, the first official release, is published.
- 2000: Python 2.0 introduces list comprehensions and a garbage collection system.
- 2008: Python 3.0, also known as Python 3000 or Py3k, is released with significant changes to the language.
- 2020: Python 2.7, the last release of Python 2, reaches end-of-life, marking the official shift to Python 3.
Frameworks and Libraries: Enhancing Python’s Power
Frameworks
- Django: A high-level web framework for rapid development, Django follows the “Don’t Repeat Yourself” (DRY) principle.
- Flask: A lightweight and modular micro-framework, Flask provides flexibility and simplicity for building web applications.
- FastAPI: Known for its high performance and ease of use, FastAPI is particularly popular for building APIs.
Libraries
- Pandas: Ideal for data manipulation and analysis, Pandas provides data structures like DataFrames.
- NumPy: The foundation for numerical computing in Python, NumPy offers support for large, multi-dimensional arrays and matrices.
- Matplotlib: For creating static, interactive, and animated visualizations, Matplotlib is a go-to library.
Features That Make Python Stand Out
- Clean and Readable Code: Python’s syntax emphasizes readability and simplicity, making it easy to write and understand code.
- Versatility: From web development to data science to machine learning, Python can handle a wide range of tasks.
- Large Community: Python has a massive and active community of developers who contribute to its libraries, frameworks, and educational resources.
- Rich Ecosystem: With thousands of third-party packages available through the Python Package Index (PyPI), developers can find tools for almost any task.
Python Compilers: Turning Code into Action
Python, as an interpreted language, runs through an interpreter that executes code line by line. However, there are tools available to compile Python code into executable files for better performance and distribution. Some popular Python compilers include:
- PyInstaller: Converts Python programs into standalone executables, under Windows, Linux, Mac OS X, FreeBSD, Solaris, and AIX.
- Nuitka: A Python compiler that translates Python code into optimized C code, which can then be compiled into an executable.
- Cython: Not a traditional compiler, but a static compiler for both the Python language and the extended Cython programming language.
Conclusion
Python is a powerful and beginner-friendly programming language that offers numerous advantages for those just starting their coding journey. Its simplicity, versatility, and strong community support make it an excellent choice for beginners and professionals alike. So why wait? Start exploring Python and unlock a world of possibilities in the exciting field of programming!
FAQs
Q: What is Python programming language used for?
A: Python is often used for web development, data analysis, artificial intelligence, scientific computing, and more.
Q: Can you provide some examples of Python applications?
A: Python is a popular language for building websites (Django), machine learning models (TensorFlow), scientific computations (NumPy), and automation scripts.
Q: What are the main features of Python programming language?
A: Python has a simple syntax, supports multiple programming paradigms like object-oriented and functional programming, and offers a wide range of libraries for diverse tasks.
Q: How does Python compare to other programming languages in terms of its history?
A: Python 2.0 was released in 2000 and since then, it has become one of the most commonly used languages in various fields due to its simplicity and versatility.
Q: What is the significance of Python libraries in programming?
A: Python libraries provide pre-written code for various tasks, saving time for developers and enabling them to build complex applications efficiently.
Q: Could you explain the relation between Python and Monty Python?
A: Python, the programming language, is not related to the British comedy group Monty Python. The name was chosen to be unique and fun.
Q: How can beginners start learning Python?
A: Beginners can start with a Python tutorial that covers the basics, such as variables, loops, and functions. There are many online resources available for free.
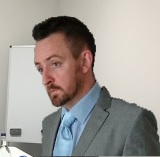
I am a self-motivated, passionate website designer and developer. I have over ten years of experience in building websites and have developed a broad skill set including web design, frontend and backend development, and SEO.
Using my growing knowledge base I have built my own company (scriptedart.co.uk) creating websites, e-commerce stores and producing custom graphics and web app functionality for a range of local businesses.