What Is Javascript (JS)
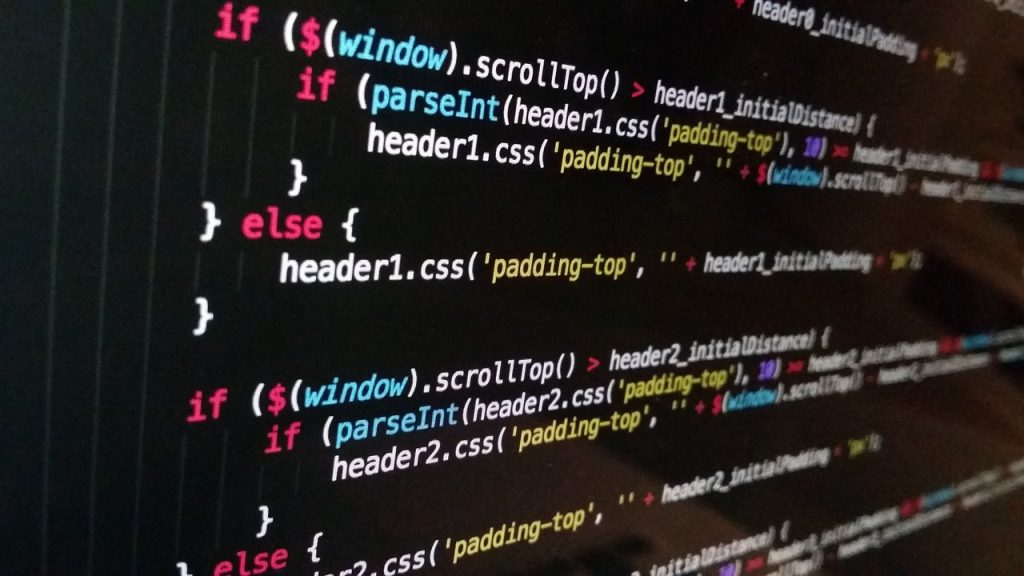
What Is Javascript (JS)?
JavaScript Tutorial : An Overview of Javascript Programming Language
Javascript, also known as JS, is a programming language that is primarily used for web development. It was created in 1995 by Brendan Eich and has since become a fundamental component of the internet. With its ability to enhance the functionality and interactivity of websites, Javascript has revolutionized the way we experience the online world. In this comprehensive guide, we will delve into the key aspects of Javascript and explore its significance in modern web development.
How Does Javascript Work?
Javascript enables developers to bring websites to life by adding dynamic features and interactivity. It is the language responsible for interactive forms, dynamic image galleries, real-time updates, and a wide array of other dynamic functionalities. Additionally, Javascript can manipulate HTML and CSS elements, allowing for responsive and intuitive designs. With its versatile nature, JS has become an indispensable tool for creating engaging and user-friendly web experiences.
How Is Javascript Used in Web Development?
One of the greatest strengths of JS is its integration within HTML and CSS. Developers can embed Javascript code directly into the HTML markup of a webpage, or they can link to external Javascript files. This seamless integration allows JS to interact with the webpage’s elements, modifying and manipulating them in real-time.
Furthermore, Javascript is extensively used in frameworks such as React.js, Angular.js, and Vue.js, which provide developers with powerful tools and libraries for building complex web applications. These frameworks leverage the flexibility and efficiency of JS to streamline the development process and create robust and scalable web solutions.
Why Learn JavaScript?
JavaScript holds immense significance in the web development domain due to its wide range of applications. From adding interactivity to web pages to creating complex web applications, JavaScript is a crucial tool for developers. Learning JavaScript will provide you with the skills to enhance user experience, build modern websites, and even create server-side applications using frameworks like Node.js.
JavaScript Basic Concepts and Syntax
JavaScript Code: A Closer Look
JavaScript code is written in a script tag either in the <head>
or <body>
section of an HTML document. Alternatively, it can also be written in an external .js
file and linked to the HTML document. Here’s an example of a JavaScript code snippet:
function greet() {
console.log("Hello, World!");
}
greet();
In this example, we define a function called greet()
that logs the message “Hello, World!” to the console. The function is then invoked using the greet()
statement.
Understanding JavaScript Variables and Data Types
JavaScript supports various data types such as strings, numbers, booleans, arrays, objects, and more. Variables are used to store and manipulate data within a program. Here’s an example:
let name = "John";
let age = 25;
let isStudent = true;
In this example, we declare three variables: name
with a string value, age
with a number value, and isStudent
with a boolean value.
Examples of Javascript Code
Here are some basic examples of JavaScript code:
1. Hello, World!
console.log("Hello, World!");
2. Variables and Data Types
// Variables
let name = "John";
let age = 25;
// Data types
let isStudent = true;
let height = 175.5;
console.log(name);
console.log(age);
console.log(isStudent);
console.log(height);
3. JavaScript Functions
// Function declaration
function greet(name) {
console.log("Hello, " + name + "!");
}
// Function call
greet("Alice");
greet("Bob");
4. Conditional Statements
let temperature = 22;
if (temperature > 30) {
console.log("It's a hot day!");
} else if (temperature >= 20 && temperature <= 30) {
console.log("It's a pleasant day.");
} else {
console.log("It's a cold day.");
}
5. Loops
// For loop
for (let i = 1; i <= 5; i++) {
console.log("Count: " + i);
}
// While loop
let counter = 0;
while (counter < 3) {
console.log("While loop: " + counter);
counter++;
}
6. Arrays
let colors = ["red", "green", "blue"];
// Accessing elements
console.log(colors[0]); // Output: red
// Adding an element
colors.push("yellow");
// Iterating through the array
for (let color of colors) {
console.log(color);
}
7. Objects
// Object literal
let person = {
name: "Jane",
age: 30,
isStudent: false,
};
// Accessing properties
console.log(person.name);
console.log(person.age);
// Adding a new property
person.gender = "female";
// Iterating through the object properties
for (let key in person) {
console.log(key + ": " + person[key]);
}
How to Add Javascript Code to HTML Content – Javascript HTML and CSS in the Web Page
Once you’re confident in your knowledge, open your HTML file and locate the tag. This is where you’ll insert your JavaScript code. Make sure to include the “src” attribute and specify the file path if you have an external JavaScript file.
If you prefer to write JavaScript directly within the HTML file, you can do so by placing the code between the opening and closing tags. Lastly, save your HTML file and open it in a web browser to see the magic happen. With JavaScript now in the mix, your website will have a dynamic edge that is sure to impress your visitors and keep them coming back for more.
<!-- index.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JavaScript Example</title>
</head>
<body>
<h1>My JavaScript Example</h1>
<!-- Using Javascript code within the page-->
<script>
// Your code can be placed between the script tags
</script>
<!-- Include the JavaScript file at the bottom of the page rather than the top to prevent render-blocking Javascript issue for page performance. If you want to use jQuery code then remember to place it after you have linked the file or the browser won't be able to read it-->
<script src="script.js"></script>
</body>
</html>
JavaScript Libraries and Frameworks
JavaScript Libraries: Enhancing Web Development
JavaScript libraries such as jQuery, React, and Vue.js provide pre-written functions and code snippets that simplify web development tasks. These libraries offer features like DOM manipulation, AJAX requests, and animation effects, saving developers time and effort when building web applications.
JavaScript Frameworks: Powering Scalable Applications
JavaScript frameworks like Angular, React, and Vue.js provide a comprehensive set of tools, patterns, and modules to build scalable and maintainable web applications. These frameworks follow the MVC (Model-View-Controller) architecture and offer features like component-based development, data binding, and state management.
Read Also :
Understanding React Framework – The Basics
Understanding Angular Framework – The Basics
A Guide to Languages and Frameworks
Limitations of JavaScript
JavaScript Limitations and Workarounds
While JavaScript is a powerful language, it does have certain limitations. For instance, JavaScript executes on the client-side, making the source code accessible to users. This exposes potential security risks. However, developers can mitigate these risks by implementing server-side JavaScript or employing security measures.
Another limitation is that JavaScript performance can vary across different browsers and devices. Code optimization and using efficient algorithms can help overcome this challenge and improve overall performance.
The Role of Javascript in Front-end Development
When it comes to front-end development, Javascript plays a vital role in creating interactive and visually appealing user interfaces. By manipulating the Document Object Model (DOM), a representation of the webpage’s structure, Javascript can dynamically change the content and layout of a webpage based on user interactions.
Moreover, Javascript enables the implementation of animations, sliders, and other interactive elements to enhance user engagement. With the help of libraries like jQuery and CSS frameworks like Bootstrap, developers can easily incorporate pre-existing Javascript functionalities into their projects, saving time and effort.
What makes Javascript great for controlling HTML Elements
JavaScript is absolutely fantastic when it comes to controlling HTML elements.
First and foremost, JavaScript allows you to dynamically modify webpage content without requiring a page reload. This means seamless user experiences and instant updates.
Furthermore, JavaScript offers a wide range of powerful methods and functions that make manipulating HTML elements a breeze. Whether you want to change the color, size, or position of an element, JavaScript has got you covered. And let’s not forget about event handling!
With JavaScript, you can effortlessly add interactivity to your web pages. From simple button clicks to complex animations, JavaScript gives you the power to engage your users like never before. So, the next time you want to take control of your HTML elements and create a dynamic and engaging web experience, JavaScript is the only way to go!
Use Javascript for Back-end Development
While Javascript initially gained popularity as a front-end programming language, it has also made significant strides in the realm of server-side development. With the advent of Node.js, a runtime environment for executing Javascript code outside of the browser, JS can now be used as a full-stack language.
Node.js allows developers to build server-side applications using Javascript, which provides greater consistency and flexibility across the entire web development stack. This opens up vast opportunities for creating real-time applications, web servers, and APIs, making JS a powerful tool for full-stack developers.
Conclusion: Harnessing the Power of Javascript
In conclusion, Javascript is a versatile and essential programming language that has transformed the landscape of web development. From enhancing user interfaces to enabling server-side applications, JS offers a wide range of functionalities that empower developers to create immersive and dynamic web experiences.
Whether you are a beginner looking to enter the world of web development or a seasoned professional seeking to expand your skillset, learning Javascript is a valuable investment. With its ubiquity and growing demand in the industry, mastering Javascript will not only enhance your career prospects but also allow you to craft exceptional web experiences that captivate and engage users.
Remember the key takeaways:
- JavaScript is a versatile and lightweight programming language used for web development.
- JavaScript libraries and frameworks streamline and enhance web development processes.
- JavaScript has certain limitations, but workarounds and optimization techniques can be applied.
- Continuously expanding your knowledge and exploring new JavaScript advancements is crucial in this ever-evolving field.
So, what are you waiting for? Start your JavaScript journey today and unlock the power to create innovative web experiences!
JavaScript FAQ’s
Q: What is JavaScript (JS)?
A: JavaScript is a programming language commonly used to create interactive effects within web browsers. It is primarily used as a client-side scripting language to enhance the functionality of web pages.
Q: How is JavaScript different from Java?
A: Despite the similar names, JavaScript and Java are entirely different languages with different use cases. JavaScript is mainly used for client-side scripting in web development, while Java is a general-purpose programming language often used for developing standalone applications and server-side code.
Q: What are the major web browsers that support JavaScript?
A: All major web browsers such as Chrome, Firefox, Safari, Edge, and Opera support JavaScript, allowing developers to write code that will run consistently across different browsers.
Q: Why do developers use JavaScript?
A: JavaScript is widely used by web and mobile developers because it enables them to create dynamic and interactive web pages. It allows for the manipulation of HTML elements, handling user events, and communicating with external web servers.
Q: Is JavaScript only used for client-side scripting?
A: No, JavaScript is not restricted to client-side scripting. It can also be used for server-side development with platforms like Node.js. This allows developers to write server-side code using JavaScript.
Q: What is the role of JavaScript in the world wide web?
A: JavaScript plays a crucial role in web development, as it is used to create engaging and interactive user experiences on websites. It is utilized to build dynamic content, validate forms, create animations, and more.
Q: How is JavaScript executed by web browsers?
A: Web browsers use a JavaScript engine to interpret and execute JavaScript code. Each browser has its own JavaScript engine, for example, Chrome uses the V8 engine, while Firefox uses SpiderMonkey.
Q: Can JavaScript be used to create machine code?
A: No, JavaScript does not produce machine code. Instead, it is executed by the JavaScript engine in the browser, translating the JavaScript code into machine code that the computer can understand and execute.
Q: What are some common uses of JavaScript for web developers?
A: Web developers use JavaScript to create interactive features such as sliders, pop-up windows, form validations, interactive maps, and responsive menus. Additionally, it is used for asynchronous communication with web servers using AJAX.
Q: Where can I find reliable information about JavaScript?
A: The Mozilla Developer Network (MDN) is considered a reliable and comprehensive resource for JavaScript documentation, tutorials, and references. It provides detailed information on all aspects of JavaScript programming.
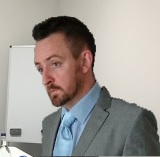
I am a self-motivated, passionate website designer and developer. I have over ten years’ experience in building websites and have developed a broad skill set including web design, frontend and backend development, and SEO.
Using my growing knowledge base I have built my own company (scriptedart.co.uk) creating websites and ecommerce stores and producing custom graphics and web app functionality for a range of local businesses.