Understanding Angular Framework – Getting Started with Angular Development

Understanding Angular: A Comprehensive Guide To This JavaScript Framework
Are you interested in learning about Angular, the popular JavaScript framework used for building web applications? Look no further! This comprehensive guide will take you through the ins and outs of Angular, helping you understand its functionality, features, and benefits. Whether you are a beginner or have some experience with web development, this article will serve as a valuable resource to enhance your understanding of Angular.
What is Angular Framework?
Angular is a versatile and powerful JavaScript framework developed and maintained by Google. It is commonly used for creating responsive, dynamic, and interactive web applications. Angular offers a comprehensive set of tools and features that simplify the development process and enhance user experience.
What’s The Difference Between Angular And AngularJS?
Angular and AngularJS may sound similar, but they have significant differences that shape their functionality.
AngularJS, also known as Angular 1, is an older version of the web development framework, whereas Angular refers to versions 2 and above.
The main contrast lies in their architecture – AngularJS operates on JavaScript, while Angular uses TypeScript, a superset of JavaScript.
This switch enhances Angular’s performance and scalability.
Additionally, Angular offers improved loading speed and better mobile support compared to AngularJS.
So, if you’re diving into web development, understanding these distinctions will help you choose the right tool for your projects and elevate your coding skills.
Why Choose Angular?
- Efficiency: Angular provides a structured approach to web development, allowing developers to write clean and maintainable code. Its modular architecture enables easy reuse of components, reducing development time and effort.
- Scalability: With Angular, you can build applications of any size and complexity. Its powerful features, such as dependency injection and modular design, ensure smooth scalability and extensibility.
- Performance: Angular leverages modern web technologies and best practices to deliver high-performance applications. It utilizes features like lazy loading, ahead-of-time (AOT) compilation, and tree shaking to optimize bundle size and improve loading speed.
- Cross-platform Development: Angular enables you to build applications that work seamlessly across multiple platforms, including desktop, mobile, and web. Its code can be easily reused to create native mobile applications using frameworks like Ionic or NativeScript.
- Dynamic Web Applications: Angular is designed for creating dynamic, single-page applications (SPAs) where user interactions are smooth and seamless.
- MVC Architecture: It follows the Model-View-Controller (MVC) architecture, making it easier to manage and scale large applications.
- Modular Development: Angular applications are built using modules, allowing for better organization and reusability of code.
- Two-Way Data Binding: Angular’s data binding synchronizes the model and the view, reducing boilerplate code.
- Extensive Ecosystem: Angular comes with a rich ecosystem of tools, libraries, and community support.
- Typescript Integration: Angular is written in TypeScript, which brings static typing and other advanced features to JavaScript development.
Getting Started with Angular
To begin your journey with Angular, you need to have a basic understanding of HTML, CSS, and JavaScript. Familiarity with TypeScript, a superset of JavaScript, is also beneficial as Angular is primarily written in TypeScript.
Let’s now explore the key components and concepts of Angular that you need to grasp before diving deeper into development.
Modules and Components
Angular applications are organized into modules, which act as containers for related components, services, and other application features. A component represents a specific part of the user interface and encapsulates its functionality, styling, and templates.
Angular Templates and Data Binding
Angular’s powerful data binding system allows you to establish a connection between the application’s data and user interface elements. With Angular’s template syntax, you can easily display and manipulate data without writing excessive JavaScript code.
Services and Dependency Injection
Services play a crucial role in Angular as they provide shared data, business logic, and utility functions. Dependency injection, a core concept of Angular, enables efficient communication between components and services.
Routing and Navigation
Angular’s built-in router allows you to handle navigation and create multiple views within your application. You can define routes and associate them with specific components, enabling users to navigate between different sections of your application seamlessly.
Angular Architecture: How Does It Work?
Angular’s architecture is based on components, which are the building blocks of an Angular application. A component in Angular is a TypeScript class with an HTML template and an optional CSS file. When you build an Angular application, you’ll work with components to create the user interface. Here’s a brief overview:
What is an Angular Component?
An Angular component is a TypeScript class that interacts with the HTML view and manages the application logic. It consists of:
- Template: This is the HTML markup that defines the component’s view.
- Class: The TypeScript class contains properties and methods that define the component’s behavior.
- Metadata: Decorators like
@Component
provide additional information about the component.
Angular Directives: Enhancing HTML with Angular
Directives in Angular are markers on a DOM element that tell Angular to do something to that element. There are three types of directives:
- Component Directives: These are the most common directives, representing reusable UI components.
- Attribute Directives: These change the appearance or behavior of an element, component, or another directive.
- Structural Directives: These alter the DOM layout by adding or removing elements.
Advantages of Angular: Why Choose Angular for Web Development?
When deciding on a framework for your next project, understanding the advantages of Angular can help you make an informed choice. Let’s explore some key benefits:
Advantages of Angular
- Rapid Development: Angular’s CLI (Command Line Interface) makes it easy to create, build, and deploy applications quickly.
- Dependency Injection: Angular has a built-in dependency injection system, making components more reusable and testable.
- Two-Way Data Binding: As mentioned earlier, Angular’s data binding ensures synchronization between the model and the view.
- Angular Material Components: Angular Material is a library of reusable and well-tested UI components based on Material Design.
- Testing Support: Angular provides robust testing support out of the box, with tools like Karma and Jasmine.
Limitations of Angular: Things to Consider
While Angular offers a plethora of advantages, it’s essential to be aware of its limitations as well. No framework is perfect, and understanding the drawbacks can help you mitigate challenges:
Limitations of Angular
- Steep Learning Curve: Angular has a relatively steep learning curve, especially for beginners.
- Performance: Large Angular applications may face performance issues if not optimized properly.
- AngularJS Migration: If you’re migrating from AngularJS to Angular, the process can be complex and time-consuming.
- Size: The size of Angular applications can be larger compared to other frameworks, affecting load times.
How to Use Angular: Practical Tips and Tricks
Now that we’ve covered the basics, let’s discuss how to start using Angular in your projects effectively.
Getting Started with Angular
- Installation: Use Angular CLI to create a new Angular project.
- Create Components: Start by creating components for different parts of your application.
- Services and Dependency Injection: Use services for reusable data logic and leverage Angular’s dependency injection.
- Routing: Implement routing to navigate between different views of your application.
- Forms: Angular provides powerful form-handling capabilities for user input validation.
- HTTP Client: Use Angular’s built-in HTTP client to communicate with backend servers.
Common Angular Commands
ng new my-app
: Create a new Angular application.ng generate component my-component
: Generate a new component.ng serve
: Run the development server.ng build
: Build the application for production.
Exploring the Angular Ecosystem
Angular’s ecosystem goes beyond the core framework. Let’s take a quick look at some popular tools and libraries in the Angular ecosystem:
Angular Ecosystem
- Angular Material: A library of UI components based on Google’s Material Design.
- RxJS: Angular heavily uses RxJS for reactive programming.
- NgRx: State management library for Angular applications, based on Redux.
- Angular Universal: Server-side rendering for Angular applications.
- Angular CLI: Command Line Interface for Angular, providing a streamlined development experience.
Conclusion: Mastering Angular Development
In conclusion, Angular is a powerful framework for building modern web applications. From its component-based architecture to its extensive ecosystem, Angular offers developers a robust toolkit for creating dynamic and responsive UIs. Remember these key points as you embark on your Angular journey:
- Angular is a JavaScript framework for building client-side web applications.
- Components are the building blocks of an Angular application, consisting of a TypeScript class, HTML template, and metadata.
- Angular provides advantages like rapid development, dependency injection, and testing support.
- Considerations include the learning curve, performance, and size of Angular applications.
- Use Angular CLI for quick project setup and explore the rich Angular ecosystem for additional tools and libraries.
See Also
FAQs
Q: What is Angular?
A: Angular is a platform and framework for building single-page client applications using HTML, CSS, and JavaScript. It is an open-source project maintained by Google and a vibrant community of developers.
Q: What are the features of Angular?
A: Angular supports features such as dependency injection, two-way data binding, reusable components, routing, forms handling, and more. It provides a comprehensive solution for building dynamic web applications.
Q: How does Angular handle dependency injection?
A: Angular uses dependency injection to provide components with the services or objects they need. This helps in keeping the components loosely coupled and allows for easier testing and reusability of code.
Q: Why do websites require JavaScript to use Angular?
A: Angular is a JavaScript framework, meaning it relies on JavaScript for its functionality. Websites using Angular need to have JavaScript enabled in order to run Angular applications properly.
Q: How does Angular CLI help in developing Angular applications?
A: Angular Command Line Interface (CLI) provides various tools and commands to streamline the development process of Angular applications. It helps in generating components, services, modules, and more, as well as optimizing the build process.
Q: What is the role of Angular developers in building Angular applications?
A: Angular developers are responsible for designing, developing, and maintaining Angular applications. They work with Angular’s components, services, modules, and other features to create dynamic and responsive web applications.
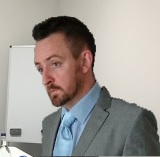
I am a self-motivated, passionate website designer and developer. I have over ten years of experience in building websites and have developed a broad skill set including web design, frontend and backend development, and SEO.
Using my growing knowledge base I have built my own company (scriptedart.co.uk) creating websites, e-commerce stores and producing custom graphics and web app functionality for a range of local businesses.