Everything You Need To Know About Ruby On Rails Framework – MVC (Model View Controller) Framework for Modern Web Apps

Understanding Ruby On Rails: A Guide to Building Web Applications
Introduction:
Are you interested in web development? Do you want to build robust and efficient web applications? Look no further than Ruby on Rails. In this article, we will dive deep into the world of Ruby on Rails and explore its power, versatility, and ease of use. Whether you’re a beginner or an experienced developer, this guide will provide you with a solid understanding of Ruby on Rails.
What is Ruby On Rails?
Ruby on Rails, commonly referred to as Rails, is a popular open-source web application framework written in Ruby. It follows the Model-View-Controller (MVC) architectural pattern, making it easy to develop and maintain web applications. With Rails, developers can quickly build applications with less code, leading to higher productivity and efficient development.
Why Learn Ruby On Rails Framework?
There are several reasons why Ruby on Rails stands out among other web development frameworks:
- Convention over Configuration: Rails follows the principle of “convention over configuration,” which means it provides sensible defaults and conventions, reducing the need for developers to make configuration choices. This leads to faster development as developers can focus on writing application-specific code rather than spending time on repetitive configurations.
- Gems and the Rails Ecosystem: Ruby on Rails has a vast collection of open-source libraries, known as gems, that provide pre-built functionalities. The Rails ecosystem is mature and well-maintained, allowing developers to leverage existing functionality and focus on building specific features without reinventing the wheel.
- Rapid Prototyping: Rails embraces the Agile development methodology, making it ideal for rapid prototyping. With Rails, developers can quickly iterate on ideas and get working prototypes up and running in a short amount of time. This agility allows for faster feedback and ultimately leads to better final products.
Getting Started with Ruby On Rails Applications
Now that we have a basic understanding of what Ruby on Rails is and why it’s a great choice for web development, let’s explore how to get started with it:
- Installing Ruby: Before diving into Rails, you need to have Ruby installed on your machine. Visit the official Ruby website and follow the installation instructions for your operating system.
- Installing Rails: Once Ruby is installed, you can easily install Rails using the command line. Open your terminal or command prompt and enter the following command:
gem install rails
. This will install the latest version of Rails. - Creating Your First Rails Application: With Rails installed, you’re ready to create your first application. Open your terminal or command prompt and navigate to the desired directory where you want to create your application. Then, run the command
rails new myapp
. This will generate a new Rails application with the name “myapp.”
How to Install Ruby on Rails
Installing Ruby on Rails is straightforward, thanks to tools like RubyGems and Bundler. Here’s a basic installation guide:
- Install Ruby: If you haven’t already, install Ruby on your system. You can use tools like RVM or rbenv for managing Ruby versions.
- Install Rails Gem: Once Ruby is installed, use the following command to install Rails:
gem install rails
- Verify Installation: After installation, verify that Rails is installed correctly by running:
rails -v
This should display the installed Rails version.
Exploring the Ruby On Rails Structure – MVC Architecture
What is MVC?
MVC stands for Model-View-Controller, a software architectural pattern widely used in web development. In the context of Ruby on Rails:
- Model: Represents the data and business logic of the application.
- View: Handles the presentation layer, rendering data to the user.
- Controller: Acts as an intermediary, processing user requests, and updating the model or view accordingly.
How Does MVC Work in Ruby on Rails?
In Ruby on Rails, the MVC pattern is central to its architecture:
- Model: Rails models are Ruby classes that interact with the application’s data. They handle database operations and business logic.
- View: Views are templates written in HTML with embedded Ruby code (ERB). They present data to the user and can be rendered dynamically.
- Controller: Controllers handle incoming requests, process parameters, and interact with models and views. They serve as the entry point for the application’s logic.
The Role of Models, Views, and Controllers
- Models: Responsible for database interactions, validations, and business logic. They are the backbone of the application’s data layer.
- Views: Present data to the user in a readable format. Views often use layouts and partials to maintain consistency across pages.
- Controllers: Handle HTTP requests, route them to the appropriate actions, and coordinate the flow of data between models and views.
Exploring Ruby on Rails Features
Creating a New Rails Application
To create a new Rails application, use the following command:
rails new myapp
This will generate the basic structure for a new Rails application, including directories for models, views, controllers, and more.
Working with Ruby Gems and Plugins
Gems and plugins are essential in extending the functionality of a Rails application. Gems are packages of reusable code, while plugins are specific to Rails.
Installing a Gem
To install a gem, add it to the Gemfile
and run bundle install
:
# Gemfile gem 'devise', '~> 4.8.0'
Using Rails Generators
Rails generators are command-line tools that create files and templates for various parts of your application. For example:
rails generate controller Welcome index
This command generates a controller with an index
action and corresponding view.
Building Web Applications with Ruby on Rails
The Rails Asset Pipeline
The Asset Pipeline in Rails is responsible for managing CSS, JavaScript, and image assets. It processes and compresses these assets for efficient delivery to the browser.
Implementing Authentication and Authorization
Authentication and authorization are critical aspects of web applications. Gems like Devise provide ready-to-use solutions for user authentication.
Testing Your Rails Application
Rails comes with built-in testing frameworks like RSpec and MiniTest. Writing tests ensures the reliability and integrity of your application.
Advanced Topics in Ruby on Rails Web Application Framework
Scaling Rails Applications
As your Rails application grows, you may need to consider scaling strategies such as caching, load balancing, and database optimization.
Integrating Frontend Frameworks
Rails works well with frontend frameworks like React or Vue.js. You can use tools like Webpacker to manage frontend assets.
Deploying a Rails Application
Deploying a Rails application to production involves setting up a web server, configuring databases, and managing environments. Services like Heroku or AWS make deployment easier.
Ruby on Rails code examples
Creating a New Rails Application
Step 1: Generate a new Rails application
Let’s create a new Rails application called blog_app
.
rails new blog_app
This command will generate the basic structure for a new Rails application.
Step 2: Navigate into the new application directory
cd blog_app
Creating a Model and Migration
Step 1: Generate a new model and migration
Let’s create a Post
model with title
and content
attributes.
rails generate model Post title:string content:text
This will generate a model file in app/models/post.rb
and a migration file in db/migrate
.
Step 2: Run the migration to create the posts
table
rails db:migrate
In the Post
model, we define that each Post
object will have a title
attribute of type string
and a content
attribute of type text
. The migration file (db/migrate/[timestamp]_create_posts.rb
) creates a posts
table with these columns.
Creating a Controller
Step 1: Generate a new controller
Let’s create a PostsController
with an index
action.
rails generate controller Posts index
This will generate a controller file in app/controllers/posts_controller.rb
and corresponding views in app/views/posts/
.
Step 2: Define the index
action in PostsController
Open app/controllers/posts_controller.rb
:
class PostsController < ApplicationController
def index
@posts = Post.all
end
end
Creating Views
Step 1: Create a view to display posts (index.html.erb
)
Open app/views/posts/index.html.erb
:
<h1>All Posts</h1>
<ul>
<% @posts.each do |post| %>
<li>
<h2><%= post.title %></h2>
<p><%= post.content %></p>
</li>
<% end %>
</ul>
- The
PostsController
has anindex
action that retrieves all posts from the database usingPost.all
. This action sets@posts
as an instance variable, which can then be accessed in the corresponding view. - The
index.html.erb
view file loops through each post in@posts
and displays itstitle
andcontent
.
Routing
Step 1: Define routes for PostsController
Open config/routes.rb
:
Rails.application.routes.draw do
resources :posts, only: [:index]
root 'posts#index'
end
This will create a route for the index
action of PostsController
and set it as the root route.
- We use the
resources :posts, only: [:index]
line to create RESTful routes for thePostsController
, specifically only for theindex
action. - The
root 'posts#index'
line sets theindex
action ofPostsController
as the root route, meaning it will be the default page when navigating to the root URL.
Creating a Seed File
Step 1: Create a seed file to populate the posts
table
Create a file db/seeds.rb
:
Post.create(title: 'First Post', content: 'This is the content of the first post.')
Post.create(title: 'Second Post', content: 'This is the content of the second post.')
# Add more posts as needed
Step 2: Run the seed file to populate the database
rails db:seed
- The seed file (
db/seeds.rb
) is used to populate the database with sample data. Here, we create two posts usingPost.create(title: '...', content: '...')
. - Running
rails db:seed
executes this file and inserts the posts into theposts
table in the database.
Running the Rails Server
Step 1: Start the Rails server
rails server
- Starting the Rails server (
rails server
) allows us to access the Rails application in the browser. - Visiting
http://localhost:3000
displays the list of posts from theindex
action ofPostsController
.
Visit http://localhost:3000
in your browser to see the list of posts.
key takeaways:
- Ruby on Rails is a powerful web framework written in Ruby, known for its developer-friendly conventions and rapid development.
- The MVC architecture in Rails separates concerns, making applications easier to maintain and scale.
- Creating a new Rails application is as simple as running a command, and Rails’ generators help scaffold components quickly.
- Gems and plugins extend Rails’ functionality, while the Asset Pipeline manages assets efficiently.
- Authentication, authorization, and testing are crucial aspects of building robust Rails applications.
- Advanced topics like scaling, integrating frontend frameworks, and deployment are essential for production-ready applications.
Everything You Need To Know About Ruby On Rails Skills
As you gain more experience with Ruby on Rails, you can explore advanced topics and techniques. Some areas you can delve into include:
- Testing: Rails provides a robust testing framework that allows you to write automated tests for your application. Learning how to write tests will help you ensure the functionality and stability of your codebase.
- Security: Understanding how to design secure applications is crucial in today’s digital landscape. Rails provides built-in mechanisms for handling security, such as protection against common web vulnerabilities like cross-site scripting (XSS) and cross-site request forgery (CSRF).
- Performance Optimization: As your application grows, optimizing its performance becomes essential. Rails offers various tools and techniques to identify and address performance bottlenecks, such as database query optimizations, caching, and background jobs.
In conclusion, Ruby on Rails provides a powerful and user-friendly framework for building web applications. Its intuitive syntax, extensive ecosystem, and emphasis on convention over configuration make it a top choice for developers worldwide. Whether you’re a beginner or an experienced developer, Ruby on Rails is worth exploring and mastering. So, why wait? Start your journey with Ruby on Rails today!
See Also
What Is Ruby – Beginners guide
FAQs
Q: What is Ruby on Rails?
A: Ruby on Rails is a web application framework written in the Ruby programming language. It follows the MVC (Model-View-Controller) architectural pattern and is designed to make web development easier and faster.
Q: How do I install Rails?
A: You can install Rails by using the command `gem install rails` in the terminal. Make sure you have Ruby installed on your system before installing Rails.
Q: What are the key features of Rails?
A: Rails offers features such as convention over configuration, RESTful routing, automated testing, and seamless database migrations. These features make it a popular choice for web developers.
Q: How can I start a new project in Rails?
A: To start a new Rails project, you can use the command `rails new project_name` in the terminal. This will create a new Rails application with the specified name.
Q: Why should I use Rails for web development?
A: Rails is known for its developer-friendly conventions and productivity. It provides built-in solutions for common web development tasks, allowing developers to focus on building features rather than dealing with configuration.
Q: What is the relationship between Ruby and Rails?
A: Ruby is the programming language on which Rails is built. Rails is a web framework written in Ruby, which means that developers use the Ruby language to write applications using the Rails framework.
Q: How can I become a Ruby on Rails developer?
A: To become a Ruby on Rails developer, you need to learn the Ruby programming language and then familiarize yourself with the Rails framework. There are many online resources and tutorials available to help you get started.
Q: Is Ruby On Rails A Good Web Framework
A: Ruby on Rails is a fantastic web framework that has gained popularity for all the right reasons! It’s known for its simplicity and elegance, making developers’ lives easier when building web applications. With its convention over configuration principle, Ruby on Rails minimizes the need for tedious setup, allowing developers to focus on crafting the actual features of their applications.
The extensive library of gems offers a wide range of tools and functionalities to enhance productivity and streamline the development process. Its strong community support and vast documentation make it beginner-friendly, enabling newcomers to ramp up quickly. Moreover, the “Don’t Repeat Yourself” (DRY) principle encourages code reusability, leading to leaner, more maintainable codebases. So, whether you’re a novice or an experienced developer, Ruby on Rails is undoubtedly a good choice for building robust, scalable web applications with a touch of elegance and efficiency.
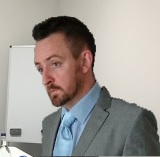
I am a self-motivated, passionate website designer and developer. I have over ten years’ experience in building websites and have developed a broad skill set including web design, frontend and backend development, and SEO.
Using my growing knowledge base I have built my own company (scriptedart.co.uk) creating websites and ecommerce stores and producing custom graphics and web app functionality for a range of local businesses.