Understanding Laravel PHP Framework – The Laravel Tutorial for Web Artisans

Understanding Laravel: A Beginner’s Tutorial to the Powerful PHP Framework
Laravel is a robust and efficient PHP framework that has gained immense popularity among web developers in recent years. It offers an elegant syntax and a wide range of features that simplify the web development process. In this beginner’s guide, we will explore the key aspects of Laravel, its benefits, and how it can be used to create powerful and scalable web applications.
What is Laravel?
Laravel is an open-source PHP framework that follows the Model-View-Controller (MVC) architectural pattern. It provides a well-structured and expressive syntax, making it easier for developers to write clean and readable code. With Laravel, developers can build web applications that are not only efficient but also maintainable and scalable.
Why Use Laravel?
Laravel offers several advantages that make it a preferred choice among developers. Firstly, it comes with a rich set of features, such as routing, caching, and authentication, which simplifies the development process. Additionally, Laravel has a large and active community, which ensures continuous support and updates. The framework also provides a comprehensive documentation that aids developers in solving any issues they may encounter.
Advantages of Laravel – Key Features of Laravel
Before we delve into the specifics, let’s first understand why Laravel is such a popular choice among developers.
- Elegant Syntax: Laravel’s syntax is simple and expressive, making it easy for developers to understand and write code. With features like method chaining and anonymous functions, Laravel allows for efficient and concise coding.
- MVC Architecture: Laravel follows the MVC architectural pattern, separating the application’s logic into three distinct layers – Model, View, and Controller. This separation of concerns promotes code reusability and maintainability.
- Database Migration: Laravel’s built-in migration system allows developers to manage database changes easily. It provides a version control system for the database, making it effortless to track and roll back changes if necessary.
- Eloquent ORM: Laravel’s Eloquent ORM (Object-Relational Mapping) simplifies database operations by providing an intuitive and fluent interface. It allows developers to interact with the database using PHP code, instead of writing SQL queries.
- Blade Templating Engine: Laravel’s Blade templating engine offers a simple and powerful way to create dynamic views. It allows for easy integration of logic within the views, making them reusable and modular.
- Authentication and Authorization: Laravel provides a robust system for user authentication and authorization. It includes features such as user registration, login, password reset, and role-based access control, making it effortless to implement secure user management systems.
Getting Started with Laravel
To begin working with Laravel, you first need to install it on your development environment. The official Laravel documentation provides detailed instructions on installation and setup. Once installed, you can start creating a new Laravel project using the command line.
Laravel also includes a powerful command-line interface known as Artisan, which offers a wide range of commands for various tasks such as generating code, migrating databases, and running tests. Familiarizing yourself with Artisan will greatly enhance your productivity as a Laravel developer.
Installing Laravel
To start working with Laravel, you’ll need to install it on your system. Here’s how you can do it:
- Composer Installation: Laravel utilizes Composer, a PHP dependency manager. Install Composer if you haven’t already.
composer global require laravel/installer
2. Create a New Laravel Project: Once Composer is installed, you can create a new Laravel project with the following command:
laravel new my-project
3. Serve Your Application: Laravel’s development server can be started with the serve
command:
php artisan serve
Using PHP In Laravel For Building Web Apps
PHP serves as the backbone for Laravel, providing a solid foundation for building robust and dynamic web applications. With Laravel’s expressive syntax and comprehensive features, you’ll find yourself manoeuvring through project development with ease and efficiency.
The flexibility and extensibility of PHP combined with Laravel’s intuitive structure make for a delightful coding experience.
Whether you’re a seasoned developer or just starting out, the vibrant community and extensive documentation surrounding PHP and Laravel ensure that help is always within reach. So, roll up your sleeves, grab your favourite caffeinated beverage, and get ready to craft some exceptional web projects using PHP for Laravel – the possibilities are endless!
Read Also ‘What Is PHP Hypertext Preprocessor’
Understanding Laravel’s Structure
Laravel follows a well-defined directory structure, which helps in organizing your application’s codebase effectively.
Directory Structure
- app: Contains the core code of your application, including models, controllers, and other PHP classes.
- config: Configuration files for various components of your application.
- routes: Defines the routes for your application’s HTTP endpoints.
- public: The web server’s document root. Public assets like CSS, JavaScript, and images are stored here.
- database: Contains migration files and seeds for database management.
- resources: Views, localization files, and assets like Sass and JavaScript.
- tests: Where you can place your PHPUnit tests.
Laravel Features in Depth
Laravel Blade Templating Engine
Blade is Laravel’s powerful templating engine, designed to make writing views simple and expressive.
Blade Syntax
Blade provides convenient shortcuts for common PHP operations. For example:
{{ $variable }}
: Outputs the contents of a variable.@if
,@else
,@elseif
: Control structures for conditional statements.@foreach
: Iterates over arrays.@include
: Includes other Blade templates.
Laravel Eloquent ORM
Eloquent ORM simplifies database operations in Laravel, providing an easy-to-use ActiveRecord implementation.
Defining Models
Models in Laravel represent database tables. You can define a model using Artisan’s make:model
command:
php artisan make:model Post
Querying with Eloquent
Eloquent allows for expressive querying:
$posts = Post::where('published', true)->orderBy('created_at', 'desc')->get();
Relationships
Eloquent makes defining and working with relationships between models straightforward:
class User extends Model
{
public function posts()
{
return $this->hasMany(Post::class);
}
}
Authentication in Laravel
Laravel provides a complete authentication system right out of the box.
Setting Up Authentication
You can set up authentication scaffolding with Artisan:
php artisan make:auth
Customizing Authentication
Laravel’s authentication system is highly customizable. You can define custom authentication guards, providers, and policies.
Advanced Laravel Techniques
Laravel Vapor
Vapor is Laravel’s serverless deployment platform, designed to simplify deploying and scaling Laravel applications.
Serverless Deployment
With Vapor, you can deploy your Laravel applications without managing servers. It automatically scales based on demand.
Laravel Ecosystem
Laravel has a thriving ecosystem with various tools and packages to enhance your development experience.
Laravel Forge
Forge provides a simple yet powerful server management and deployment tool for Laravel applications.
Laravel Mix
Mix simplifies asset compilation and management using Webpack. It’s the perfect tool for managing JavaScript and CSS assets in your Laravel projects.
Some Useful Code Examples For Laravel Developers (CRUD)
Creating a Model and Migration
Step 1: Generate a new model and migration
First, let’s create a model for a Post
and its corresponding migration file.
php artisan make:model Post -m
This command will create a Post
model in app/Models/
directory and a migration file in database/migrations/
.
Step 2: Define the posts
table schema in the migration file
Open the generated migration file (timestamp will vary) in database/migrations/
:
// database/migrations/[timestamp]_create_posts_table.php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreatePostsTable extends Migration
{
public function up()
{
Schema::create('posts', function (Blueprint $table) {
$table->id();
$table->string('title');
$table->text('content');
$table->timestamps();
});
}
public function down()
{
Schema::dropIfExists('posts');
}
}
Step 3: Run the migration to create the posts
table
Run the migration to create the posts
table in the database:
php artisan migrate
Using Eloquent ORM to interact with the Post
Model
Step 1: Create a new Post
instance
Now that the Post
model is defined, we can create a new post in our controller:
use App\Models\Post;
public function createPost()
{
$post = new Post();
$post->title = 'My First Post';
$post->content = 'This is the content of my first post.';
$post->save();
return response()->json(['message' => 'Post created successfully']);
}
Step 2: Retrieve all posts
To retrieve all posts from the database:
use App\Models\Post;
public function getAllPosts()
{
$posts = Post::all();
return response()->json($posts);
}
Step 3: Update a post
To update an existing post:
use App\Models\Post;
public function updatePost($id)
{
$post = Post::find($id);
if (!$post) {
return response()->json(['message' => 'Post not found'], 404);
}
$post->title = 'Updated Title';
$post->content = 'Updated content goes here.';
$post->save();
return response()->json(['message' => 'Post updated successfully']);
}
Step 4: Delete a post
To delete a post:
use App\Models\Post;
public function deletePost($id)
{
$post = Post::find($id);
if (!$post) {
return response()->json(['message' => 'Post not found'], 404);
}
$post->delete();
return response()->json(['message' => 'Post deleted successfully']);
}
Using Blade Templating Engine for Views
Step 1: Create a Blade template
Let’s create a simple Blade template for displaying posts:
// resources/views/posts.blade.php
<!DOCTYPE html>
<html>
<head>
<title>Posts</title>
</head>
<body>
<h1>All Posts</h1>
<ul>
@foreach($posts as $post)
<li>{{ $post->title }}</li>
@endforeach
</ul>
</body>
</html>
Step 2: Pass data to the Blade view from a Controller
In your controller, pass data to the Blade view:
use App\Models\Post;
public function showPosts()
{
$posts = Post::all();
return view('posts', ['posts' => $posts]);
}
This will render a list of post titles in the posts.blade.php
view.
These examples cover basic CRUD operations with Laravel using Eloquent ORM and how to render views using the Blade templating engine. Remember to adjust namespaces and paths according to your project structure.
Conclusion
Laravel is not just a framework; it’s a powerful tool that empowers web artisans to build robust and scalable web applications. With its elegant syntax, modular structure, and rich feature set, Laravel has become the go-to choice for many developers.
In this guide, we’ve explored the fundamentals of Laravel, from its installation and basic structure to its advanced features like Blade templating, Eloquent ORM, and authentication. We’ve also touched on advanced techniques such as Laravel Vapor and the broader Laravel ecosystem.
Whether you’re a seasoned Laravel developer or just starting, mastering Laravel opens up a world of possibilities for building modern web applications. So roll up your sleeves, dive into the Laravel documentation, and start crafting your next web masterpiece!
Key Takeaways
- Laravel is an open-source PHP framework known for its elegant syntax and powerful features.
- The framework follows the MVC architectural pattern, making it easy to build and maintain web applications.
- Features like Blade templating, Eloquent ORM, and built-in authentication simplify development tasks.
- Advanced techniques like Laravel Vapor and the Laravel ecosystem provide additional tools for deployment and development.
FAQs
Q: What is Laravel?
A: Laravel is a PHP web application framework known for its expressive syntax and elegant structure, making it a popular choice for web artisans.
Q: What are the key features of Laravel?
A: Laravel includes various features such as security enhancements, built-in tools for web application development, and an expressive syntax that simplifies coding.
Q: How does Laravel compare to other PHP frameworks?
A: Laravel is often compared to other popular PHP frameworks like Symfony and CodeIgniter for its robust features, ease of use, and continuous updates to keep up with modern web development trends.
Q: What are the components of Laravel?
A: Laravel consists of various components such as Eloquent ORM, routing, authentication, and middleware, which together provide a solid foundation for building web applications.
Q: Is Laravel suitable for beginners?
A: Yes, Laravel is a beginner-friendly framework with comprehensive documentation, vibrant community support, and a wide range of tutorials available for those who are new to web development.
Q: What sets Laravel apart as a new framework?
A: Laravel distinguishes itself as a new framework with its modern features, intuitive syntax, and commitment to staying up-to-date with the latest web development practices.
Q: How does Laravel make web development easier?
A: Laravel makes web development easier by providing developers with tools like Blade templating engine, Artisan command-line interface, and pre-built authentication scaffolding for faster project setup.
See Also
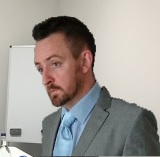
I am a self-motivated, passionate website designer and developer. I have over ten years’ experience in building websites and have developed a broad skill set including web design, frontend and backend development, and SEO.
Using my growing knowledge base I have built my own company (scriptedart.co.uk) creating websites and ecommerce stores and producing custom graphics and web app functionality for a range of local businesses.