What is PHP Programming Language (PHP Hypertext Preprocessor)
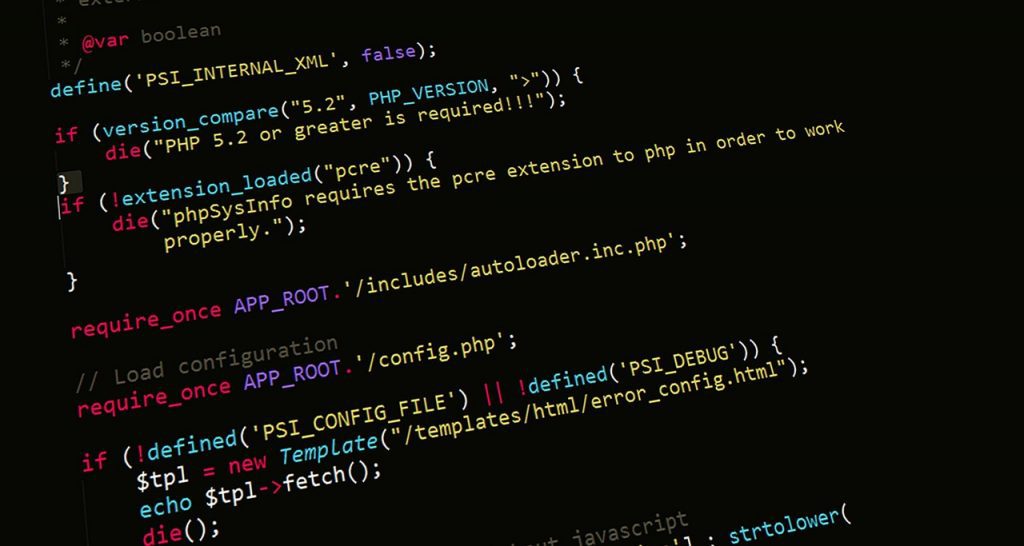
What Is PHP for Beginners?
Introduction
If you are new to web development, you may have come across the term “PHP” quite often. In this article, we will dive into the world of PHP and explore what it is and how it can be beneficial for beginners. Whether you are aspiring to become a professional web developer or simply want to understand the basics, this guide will provide you with the necessary information to get started.
Understanding PHP Server-Side Scripting
PHP, which stands for Hypertext Preprocessor, is a server-side scripting language widely used for web development. It is a powerful tool that enables developers to create dynamic websites and web applications. PHP is an open-source language, meaning it is available for anyone to use and modify freely.
PHP: A General-Purpose Scripting Language
PHP, which stands for Hypertext Preprocessor, is a server-side scripting language used primarily for web development. It was created by Rasmus Lerdorf in 1994 and has since evolved into a versatile language supporting a wide range of applications. Initially designed for handling simple tasks like form processing, PHP has grown into a full-fledged programming language capable of creating dynamic web pages, handling databases, and much more.
Why PHP Hypertext Preprocessor?
1. Versatility
PHP is a general-purpose language, meaning it can handle a variety of tasks. Whether you’re building a simple website or a complex web application, PHP provides the tools needed to get the job done.
2. Community and Support
With a vast community of developers, PHP benefits from continuous updates, security patches, and a wealth of online resources. This support network makes it easier to find solutions to problems and stay up-to-date with best practices.
3. Integration
PHP works seamlessly with databases like MySQL, making it an excellent choice for database-driven web applications. Its compatibility with other technologies and frameworks further enhances its appeal.
4. Efficiency
Being a server-side scripting language, PHP executes on the server before sending the result to the client’s browser. This reduces the load on client-side resources, resulting in faster page load times and a smoother user experience.
Learning and Using PHP Programming Language
Getting Started with PHP
If you’re new to PHP, getting started is straightforward. All you need is a text editor and a web server with PHP installed. Many web hosting services offer PHP support, making it easy to set up a development environment.
Learning Resources
There are abundant resources available for learning PHP, from online tutorials to official documentation. Websites like PHP.net provide a comprehensive guide to the language, including code examples and explanations.
PHP Community
Joining PHP communities, forums, and social media groups is a great way to connect with other developers, ask questions, and stay updated on the latest trends and practices in PHP development.
PHP Example Code
Basic PHP Script
<?php
// This is a basic PHP script that echoes "Hello, World!" to the browser.
echo "Hello, World!";
?>
- This script uses the
echo
statement to output the text “Hello, World!” to the browser. - The
<?php ?>
tags indicate the start and end of PHP code.
Variables and Concatenation
<?php
// Define variables
$name = "Alice";
$age = 30;
// Concatenate variables within a string
echo "Hello, my name is " . $name . " and I am " . $age . " years old.";
?>
- This script demonstrates variable assignment and string concatenation.
- The variables
$name
and$age
are defined with values. - The
.
operator is used to concatenate the variables within theecho
statement.
Conditional Statement
<?php
// Define a variable
$score = 85;
// Check if score is greater than or equal to 60
if ($score >= 60) {
echo "You passed!";
} else {
echo "You did not pass.";
}
?>
- This script uses an
if...else
statement to check if a student’s score is greater than or equal to 60. - If the condition is true (
$score >= 60
), it echoes “You passed!” - Otherwise, it echoes “You did not pass.”
Looping with foreach
<?php
// Define an array of fruits
$fruits = array("Apple", "Banana", "Orange");
// Loop through the array and echo each fruit
foreach ($fruits as $fruit) {
echo $fruit . "<br>";
}
?>
- This script demonstrates a
foreach
loop to iterate through an array of fruits. - For each iteration, it echoes the value of the
$fruit
variable followed by a line break (<br>
).
Function with Parameters
<?php
// Define a function to calculate the area of a rectangle
function calculateRectangleArea($length, $width) {
$area = $length * $width;
return $area;
}
// Call the function with arguments and echo the result
$rectangleLength = 5;
$rectangleWidth = 10;
$area = calculateRectangleArea($rectangleLength, $rectangleWidth);
echo "The area of the rectangle is: " . $area;
?>
- This script defines a function
calculateRectangleArea()
that takes two parameters:$length
and$width
. - Inside the function, it calculates the area of a rectangle using the formula
$length * $width
and returns the result. - The function is called with arguments
$rectangleLength
and$rectangleWidth
, and the result is echoed.
Include PHP File
<?php
// Include an external PHP file
include 'external.php';
// Call a function from the included file
echo "The result of the calculation is: " . multiply(5, 3);
?>
- This script includes an external PHP file named
external.php
. - The
include
statement allows the script to use functions and code from the included file. - In this example, it calls the
multiply()
function fromexternal.php
and echoes the result.
Error Handling with Try…Catch
<?php
// Define a function to divide two numbers
function divide($numerator, $denominator) {
if ($denominator == 0) {
throw new Exception("Division by zero.");
}
return $numerator / $denominator;
}
// Try dividing and catch any exceptions
try {
echo divide(10, 2); // Valid division
echo "<br>";
echo divide(10, 0); // This will throw an exception
} catch (Exception $e) {
echo "Caught exception: " . $e->getMessage();
}
?>
- This script defines a
divide()
function that divides two numbers. - Inside the function, it checks if the
$denominator
is zero and throws an exception if it is. - The
try...catch
block is used to attempt a division operation and catch any exceptions that may occur. - The script first successfully divides 10 by 2 and then attempts to divide 10 by 0, which triggers the exception.
Using MySQLi for Database Operations
<?php
// Database connection parameters
$servername = "localhost";
$username = "username";
$password = "password";
$database = "mydatabase";
// Create connection
$conn = new mysqli($servername, $username, $password, $database);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// SQL query
$sql = "SELECT id, firstname, lastname FROM users";
$result = $conn->query($sql);
// Check if there are rows in the result
if ($result->num_rows > 0) {
// Output data of each row
while($row = $result->fetch_assoc()) {
echo "ID: " . $row["id"]. " - Name: " . $row["firstname"]. " " . $row["lastname"]. "<br>";
}
} else {
echo "0 results";
}
// Close connection
$conn->close();
?>
- This script demonstrates using MySQLi (MySQL Improved) for database operations.
- It establishes a connection to a MySQL database using the
$servername
,$username
,$password
, and$database
variables. - An SQL query is executed to select data from a table named
users
. - If there are rows in the result, it loops through each row and outputs the ID, firstname, and lastname.
- Finally, the database connection is closed using
$conn->close()
.
History of PHP
PHP, also known as “Personal Home Page,” started its journey in 1994 under the creative hands of developer Rasmus Lerdorf. Originally intended to track visitors to his online resume, PHP quickly evolved into a robust server-side scripting language that powered dynamic websites worldwide. With the release of PHP 3 in 1998, the language transitioned into a powerful tool for web development. Over the years, PHP has continued to grow and adapt, with regular updates and improvements keeping it relevant in the ever-changing landscape of the internet. Despite some bumps along the way, the PHP community’s collaborative spirit and dedication have helped shape it into the versatile language it is today, empowering countless developers to create innovative web applications with ease.
PHP Versions
PHP 1.0 (June 8, 1995)
- Initial release.
- Basic functionality for form processing, database access, and more.
- Introduced the concept of a server-side scripting language.
PHP 2.0 (November 1997)
- Added support for dynamic web pages.
- Formatted text, simple conditionals, and basic looping.
- Support for databases like mSQL and MySQL.
PHP 3.0 (June 6, 1998)
- Major rewrite.
- Added support for more web servers, including Apache.
- Introduction of object-oriented programming (OOP) concepts.
- Better performance and stability.
PHP 4.0 (May 22, 2000)
- Major overhaul.
- Improved support for OOP with classes and objects.
- Added the Zend Engine for better performance.
- Introduced more advanced features like interfaces, exceptions, and static methods.
PHP 5.0 (July 13, 2004)
- Significant update.
- Introduction of the PHP Data Objects (PDO) extension for database access.
- Enhanced OOP support with new features like visibility (public, private, protected), abstract classes, and interfaces.
- Added the SimpleXML extension for easier XML parsing.
- Improved error handling with exceptions.
- Introduced the Zend Engine 2.
PHP 5.3 (June 30, 2009)
- Added namespaces for better organizing code.
- Introduced late static binding.
- Improved performance and reduced memory usage.
- Added the “goto” operator.
PHP 5.4 (March 1, 2012)
- Added short array syntax (
[]
) and short open tag (<?=
) for echo. - Improved performance and reduced memory footprint.
- Traits introduced for code reusability.
- Built-in web server for local development.
PHP 5.5 (June 20, 2013)
- Introduced the
finally
keyword for exception handling. - Added the
yield
keyword for generators. - Improved support for password hashing.
- Added the
password_hash()
andpassword_verify()
functions.
PHP 5.6 (August 28, 2014)
- Improved syntax and security features.
- Added constant scalar expressions.
- Introduced the
...
operator for argument unpacking. - Added the
use
operator for importing functions and constants from namespaces. - Improved performance with the Zend OPcache extension.
PHP 7.0 (December 3, 2015)
- Major release with significant improvements.
- Improved performance with the Zend Engine 3.0.
- Introduces scalar type declarations and return type declarations.
- Added the
null coalescing operator
(??
) and thespaceship operator
(<=>
). - Improved error handling with the
Throwable
interface. - Introduced anonymous classes and closure improvements.
- Support for more accurate type declarations, including
int
,float
,bool
, andstring
.
PHP 7.1 (December 1, 2016)
- Introduced nullable types (
?Type
) for parameters and return types. - Added the
void
return type. - Improved syntax with the iterable pseudo-type.
- Added the
list()
reference assignment. - Added the
iterable
type hint for parameters and return types.
PHP 7.2 (November 30, 2017)
- Improved performance and reduced memory usage.
- Added the
object
type hint. - Introduced the
Traversable
interface. - Added the
json_encode()
andjson_decode()
options for handling JSON. - Improved security with Libsodium for encryption and decryption.
PHP 7.3 (December 6, 2018)
- Added flexible heredoc and nowdoc syntax.
- Introduced trailing commas in function calls.
- Added
is_countable()
function. - Improved performance with opcache enhancements.
- Introduced the
array_key_first()
andarray_key_last()
functions.
PHP 7.4 (November 28, 2019)
- Added typed properties and class properties.
- Introduced arrow functions.
- Added the
Null Coalescing Assignment Operator
(??=
). - Introduced
preloading
for better performance. - Improved error messages and warnings.
PHP 8.0 (November 26, 2020)
- Major release with many new features.
- Introduced
Named Arguments
for function calls. - Added
Attributes
(also known as annotations). - Introduced
Union Types
andMatch Expressions
. - Added the
str_contains()
,str_starts_with()
, andstr_ends_with()
functions. Nullsafe Operator
(?->
) for null checking in chains.
What’s New in PHP?
PHP 7
One of the most significant updates to PHP was the release of version 7. This update brought numerous improvements, including increased performance, reduced memory consumption, and new language features like scalar type declarations and return type declarations.
PHP 8.0 and Beyond
Continuing the trend of innovation, PHP 8.0 introduced JIT (Just-In-Time) compilation, further boosting performance. With each new version, the PHP development team continues to refine the language, adding features that improve developer productivity and application efficiency.
PHP in Action: PHP Use Cases and Advantages
Server-Side Scripting
PHP shines as a server-side scripting language. When a user requests a PHP file from a web server, the server processes the PHP code and sends the output (usually HTML) to the user’s web browser. This dynamic process allows for the creation of interactive and personalized web experiences.
Web Development
PHP is the backbone of many popular content management systems (CMS) like WordPress, Joomla, and Drupal. These platforms leverage PHP’s capabilities to create customizable and feature-rich websites.
Form Handling
Handling form data is a common task in web development, and PHP excels in this area. Whether it’s user registration, contact forms, or search functionality, PHP provides robust tools for processing and validating form submissions.
Database Interaction
PHP’s integration with databases like MySQL makes it a powerful tool for managing data. From simple queries to complex database-driven applications, PHP’s database support is a cornerstone of its versatility.
Advantages of PHP
1. Easy to Learn
PHP’s syntax is similar to other programming languages like C and Java, making it accessible to beginners. The learning curve is gentle, allowing new developers to start building web applications quickly.
2. Open Source
PHP is open-source, meaning it’s free to use and constantly evolving thanks to contributions from developers worldwide. This open nature fosters innovation and ensures PHP remains relevant in the ever-changing landscape of web development.
3. Cross-Platform Compatibility
Whether you’re running PHP on a Linux, macOS, or Windows server, it performs consistently across different operating systems. This flexibility makes it a top choice for developers working in diverse environments.
4. Extensive Library Support
PHP has a vast standard library and a plethora of third-party libraries and frameworks. These resources streamline development, allowing developers to focus on building features rather than reinventing the wheel.
Advanced PHP Techniques
Object-Oriented Programming (OOP)
PHP supports OOP principles, allowing developers to create reusable and modular code. Classes, objects, and inheritance in PHP facilitate the building of complex applications with organized and maintainable codebases.
PHP Frameworks
Frameworks like Laravel, Symfony, and CodeIgniter provide a structured way to build web applications with PHP. These frameworks come with built-in features, such as authentication, routing, and templating, speeding up development and ensuring best practices.
PHP and MySQL
The combination of PHP and MySQL is a powerful duo for web development. PHP can connect to MySQL databases, execute queries, and handle result sets seamlessly, making it an ideal choice for applications requiring data storage and retrieval.
Conclusion: Embracing the Power of PHP
PHP continues to be a dominant force in web development, powering a vast number of websites and applications. Its versatility, performance, and extensive community support make it a valuable skill for developers of all levels. Whether you’re interested in building dynamic websites, interacting with databases, or exploring advanced programming techniques, PHP has something to offer.
In conclusion, PHP’s role as a server-side scripting language, its integration with databases, and its support for web development frameworks make it a compelling choice for developers. Whether you’re a beginner learning the basics or an experienced programmer exploring advanced techniques, PHP remains a cornerstone of modern web development.
Key Takeaways
- PHP, or Hypertext Preprocessor, is a versatile server-side scripting language used for web development.
- Advantages of PHP include its ease of learning, open-source nature, cross-platform compatibility, and extensive library support.
- PHP’s role in web development includes form handling, database interaction, and integration with frameworks like Laravel and Symfony.
- PHP 7 introduced significant improvements in performance and new language features.
- Learning PHP is accessible through online tutorials, documentation, and active community engagement.
Next time you encounter a dynamic website, chances are PHP is working behind the scenes, handling form submissions, interacting with databases, and creating the dynamic content you see. Embracing PHP opens doors to a world of web development possibilities, making it a skill worth mastering in today’s digital landscape.
FAQs
Q: What is PHP Hypertext Preprocessor?
A: PHP Hypertext Preprocessor, commonly known as PHP, is a scripting language that is especially suited for web programming. It can be used for command-line scripting and developing desktop applications. PHP is a server-side scripting language that processes the PHP page through the server before it is sent to the browser.
Q: How can PHP be used in form handling?
A: PHP can be used to handle form data submitted from HTML forms. The PHP code can access the form data, process it, validate it, and send the results back to the user.
Q: What are the major operating systems that support PHP?
A: PHP is available for most major operating systems including Microsoft Windows, macOS, Linux, and Unix.
Q: What are some features of PHP?
A: PHP is known for its flexibility and can also support a graphical user interface. It is widely used for web programming and can also be used for many programming tasks beyond web development.
Q: How does PHP handle source code?
A: PHP is a parser that reads and interprets the source code written in the PHP language. It then executes the code and produces the desired output.
Q: Is PHP still actively developed?
A: Yes, PHP is still actively developed with regular updates and new releases. The latest release is PHP 8.3 which brings new features and improvements to the language.
Q: What are the different versions of PHP available?
A: PHP has evolved over the years, with different versions released to add new features, improve performance, and fix bugs. Developers can choose to work with different PHP versions based on their project requirements.
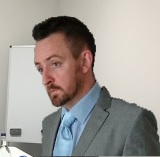
I am a self-motivated, passionate website designer and developer. I have over ten years of experience in building websites and have developed a broad skill set including web design, frontend and backend development, and SEO.
Using my growing knowledge base I have built my own company (scriptedart.co.uk) creating websites, e-commerce stores and producing custom graphics and web app functionality for a range of local businesses.