Getting Started with Django Framework: The Python Framework for Web Development
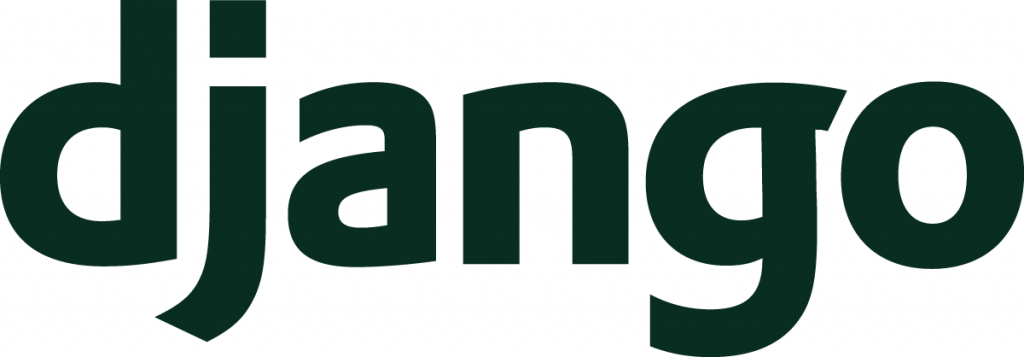
Understanding Python Django Framework: A Comprehensive Tutorial
Introduction
Django is a high-level web framework that enables developers to build web applications rapidly using Python. It follows the Model-View-Controller (MVC) architectural pattern and provides a robust toolkit for handling various web development tasks, including API development and the creation of a web app. With its clean design and efficient development methodologies, Django has gained immense popularity among developers worldwide. In this article, we will dive deep into the Django framework and explore its key features, benefits, and use cases.
What is Django?
Django is a free and open source web framework that simplifies the process of building web applications. It was developed by experienced programmers at the Lawrence Journal-World newspaper and was released publicly in 2005. Since then, Django has become one of the go-to frameworks for web development due to its scalability, security, and versatility.
Key Features of Django
- Object-Relational Mapping (ORM): Django provides a powerful ORM that allows users to interact with databases using Python objects. It simplifies database management and eliminates the need for writing complex SQL queries.
- Admin Interface: Django comes with a built-in admin interface that enables developers to manage application data, handle user authentication, and perform content management tasks without writing additional code.
- URL Routing: Django’s URL routing mechanism allows developers to map URLs to specific views, enabling clean and user-friendly URLs for improved search engine optimization (SEO) and user experience.
- Templating Engine: Django’s templating engine provides a convenient way to separate the presentation layer from the business logic. It allows developers to create reusable templates in Django are a powerful feature for customizing the user interface of a web app with HTML templates. and dynamically render data within them.
- Security: Django incorporates various security measures by default, such as protection against common web vulnerabilities like cross-site scripting (XSS), cross-site request forgery (CSRF), and SQL injection.
- Scalability: Django’s modular design and scalability make it ideal for building complex and large-scale web applications. Its ability to handle high traffic and heavy workloads ensures optimal performance even under demanding circumstances.
Benefits of Using Django Web Framework
- Rapid Development: Django’s design philosophy emphasizes rapid development and clean, pragmatic design, making it ideal for starting a new web app project. Django’s emphasis on DRY (Don’t Repeat Yourself) principle and reusable components enables talented django developers to build applications quickly, significantly reducing development time.
- Community Support: Django has a vibrant and active community of developers who contribute to its continuous improvement. This community-driven approach ensures regular updates, bug fixes, and a vast range of available libraries and packages.
- Versatility: Django can be used to build a wide range of web applications, from simple content management systems to complex e-commerce platforms. Its flexibility allows developers to customize and extend the framework according to their specific requirements.
- Maintainability: Django’s clean and organized code structure promotes code readability and maintainability. The framework’s built-in testing tools and comprehensive documentation further facilitate application maintenance and troubleshooting.
- Compatibility: Django is compatible with various databases, including PostgreSQL, MySQL, SQLite, and Oracle. It also integrates seamlessly with other Python libraries and frameworks, making it a versatile choice for developers.
Use Cases of Django
- Content Management Systems (CMS): Django’s framework is effectively utilized in the rapid development of CMS, thanks to features like the Django admin site. Django’s framework is effectively utilized in the rapid development of CMS, thanks to features like the Django admin site. Django’s admin interface and ease of content management make it an ideal choice for developing robust CMS platforms like Wagtail and Mezzanine.
- E-commerce Websites: Django’s scalability and security features make it suitable for building e-commerce websites with complex product catalogs, shopping carts, and payment gateways.
- Social Networking Sites: Django’s ability to handle large user bases and its built-in user authentication system make it suitable for developing social networking platforms.
- Data Analysis and Visualization: Django can be used as a backend framework for building data analysis and visualization tools, integrating with libraries like NumPy and Pandas.
- API Development: Django, being a high-level Python web framework, enables the seamless creation of APIs for web apps. Django, being a high-level Python web framework, enables the seamless creation of APIs for web apps. Django REST Framework, an extension of Django, provides a powerful toolkit for building APIs, making it a popular choice among developers for developing web services and mobile applications.
Getting Started with Django
Installing Django
To install Django, you can use pip, Python’s package installer. Open your terminal or command prompt and enter:
pip install django
Creating Your First Django App
Once Django is installed on your web server, you can create a new project with the following command:
django-admin startproject myproject
This will create a new directory called myproject
with the basic structure for a Django project.
Running the Development Server
Navigate into your project directory and start the development server:
cd myproject
python manage.py runserver
Now, you can visit http://localhost:8000
in your web browser to see the default Django welcome page.
Django Architecture: MVC vs. MTV
Understanding Django’s Architecture
Django follows the MTV (Model-Template-View) architectural pattern, which is similar to MVC (Model-View-Controller).
- Model: Represents the data structure. Django models define the database schema.
- Template: Handles the presentation layer, generating HTML dynamically.
- View: Controls the logic and processes requests. Views fetch data from the database using models and pass it to templates for rendering.
How Django Handles Requests
When a user sends a request to a Django-powered website:
- The URL dispatcher matches the URL to a view.
- The view processes the request, possibly interacting with the database through models.
- The view then passes data to a template for rendering.
- The rendered HTML is sent back to the user’s browser.
Django Models and ORM
Working with Models
Django models define the structure of your data. Here’s an example of a simple model for a blog post, demonstrating how to use the Django include and links features effectively.
from django.db import models
class Post(models.Model):
title = models.CharField(max_length=200)
content = models.TextField()
date_posted = models.DateTimeField(auto_now_add=True)
Django Admin Interface
With Django’s admin interface, you can manage your application’s data without writing custom views. To register the Post
model:
from django.contrib import admin
from .models import Post
admin.site.register(Post)
Templates and Views in Django
Creating Templates
Django’s template system allows you to create dynamic HTML pages. Templates are stored in the templates
directory of your app. Here’s an example template for a blog post:
<!-- blog/post_detail.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<title>{{ post.title }}</title>
</head>
<body>
<h1>{{ post.title }}</h1>
<p>{{ post.content }}</p>
</body>
</html>
Views and URL Routing
Views in Django are Python functions or classes that receive web requests and return web responses. Here’s a simple view for displaying a blog post:
from django.shortcuts import render
from .models import Post
def post_detail(request, pk):
post = Post.objects.get(pk=pk)
return render(request, 'blog/post_detail.html', {'post': post})
URL Routing
You can map URLs to views using Django’s URL dispatcher, a key feature for creating efficient Django links within your web app. Here’s an example URL configuration:
# myproject could utilize various Django modules to enhance its functionality as a web app./urls.py
from django.urls import path
from blog import views
urlpatterns = [
path('post/<int:pk>/', views.post_detail, name='post_detail'),
]
Use Django For Web Development
Django Web Development
Django is a popular choice for web development due to its:
- Security Features: Protects against common web vulnerabilities.
- Versatility: Used for a wide range of projects, from simple websites to complex web applications.
- Community and Resources: A large community of developers and extensive documentation make learning Django easier.
Examples of Django Websites
One example of a Django-built website is Instagram, which harnesses Django’s scalability and robust security features to handle millions of users worldwide effortlessly.
Furthermore, The Washington Post website also runs on Django, highlighting its flexibility in handling high traffic volumes while providing responsive and dynamic content delivery.
These websites demonstrate how Django can be utilized to create visually appealing and user-friendly platforms that cater to diverse needs. Whether it’s for social media or news publication, Django continues to empower developers to craft innovative and reliable websites that leave a lasting impact on users.
Conclusion
Understanding the Django framework is crucial for any developer looking to build dynamic and scalable web applications efficiently. Its extensive feature set, community support, and versatility make it an excellent choice for a wide range of web development projects. By leveraging Django’s powerful tools and best practices, developers can streamline their workflows and deliver high-quality applications with ease.
See Also
What Is Python Programming Language, written in Python, and how it’s utilized in Django development.
FAQs
Q: What is Django and why is it used?
A: Django is a high-level Python web framework that encourages rapid development and clean, pragmatic design. It is widely used by web developers to build web applications quickly and efficiently.
Q: How can I start learning Django as a beginner developer?
A: To begin learning Django, you can follow online tutorials specifically designed for beginners. These tutorials will walk you through creating your first Django app and understanding the basics of Django development.
Q: What are Django templates and how are they used?
A: Django templates are HTML files that contain static parts of a web page as well as template tags which generate dynamic content. They are used to create the user interface of a Django web application.
Q: How can I include templates in my Django project?
A: To include templates in your Django project, you can use the “{% include %}” template tag in your HTML files. This allows you to reuse template code across different pages of your application.
Q: Can I delete a Django app from my project?
A: Yes, you can delete a Django app from your project by removing the app folder from your project directory and unregistering it from the project’s settings file.
Q: What are the building blocks of a Django application?
A: The building blocks of a Django application include models, views, templates, URLs, and static files. These components work together to create a fully functional web application using Django.
Q: How can I use Django for my web development project?
A: You can use Django for your web development project by installing the Django framework, creating a new Django project, defining models and views, setting up URLs, creating templates, and running the development server.
Q: What is the Django software foundation and its role in the Django community?
A: The Django Software Foundation is a non-profit organization that maintains and promotes the Django web framework. It oversees the development of Django, organizes sprints and conferences, and supports the Django community.
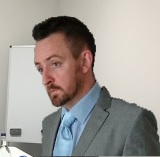
I am a self-motivated, passionate website designer and developer. I have over ten years of experience in building websites and have developed a broad skill set including web design, frontend and backend development, and SEO.
Using my growing knowledge base I have built my own company (scriptedart.co.uk) creating websites, e-commerce stores and producing custom graphics and web app functionality for a range of local businesses.